2014
Feb
03
建立一般按鈕
建立按鈕的第一步,首先你要先給按鈕一個 「x , y」 座標,再給他一個長,寬,所以我使用 CGRectMake, X = 10 , Y = 40 , Width = 100 , Height = 30 。
再來就宣告 UIButton ,接著我設定按鈕的標題與顏色,給予白色背景與黑色文字。
- setTitle 設定按鈕標題。
- setBackground 設定背景色。
- setTitleColor 設定文字顏色。
Simple Button
- CGRect buttonFrame = CGRectMake( 10, 40, 100, 30 );
- UIButton *button = [[UIButton alloc] initWithFrame: buttonFrame];
- [button setTitle:@"button" forState:UIControlStateNormal];
- [button setBackgroundColor:[UIColor whiteColor]];
- [button setTitleColor:[UIColor blackColor] forState:UIControlStateNormal];
- [view addSubview:button];
DEMO
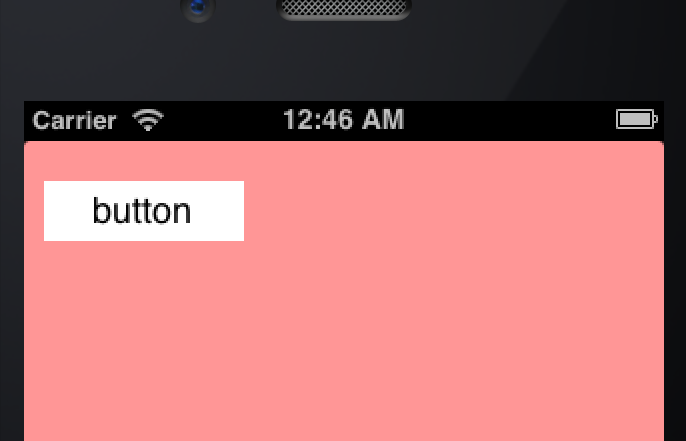
按鈕狀態 forState
forState 是按鈕狀態的設定,目前 IOS 有提供六種狀態讓我們可以切換。
- UIControlStateNormal : 一般正常狀態。
- UIControlStateSelected : 按鈕被選取後的狀態,使用 button.selected = YES 可以將按鈕切換至此狀態。
- UIControlStateApplication
- UIControlStateDisabled :使用 button.disabled = YES 可以將按鈕切換至此狀態。
- UIControlStateHighlighted:使用 button.hightlighted = YES 可以將按鈕切換至此狀態。
- UIControlStateReserved
按鈕邊框 Border
要修改按鈕的邊框,必須使用 button.layer.borderColor , button.layer.borderWidth。
使用 Border 功能前,在載入 header 的地方,要加入 QuartzCore.h。
header
- #import <QuartzCore/QuartzCore.h>
設定 Border 的 Source code 範例如下
border
- button.layer.borderColor = [[UIColor blackColor] CGColor];
- button.layer.borderWidth = 2;
DEMO
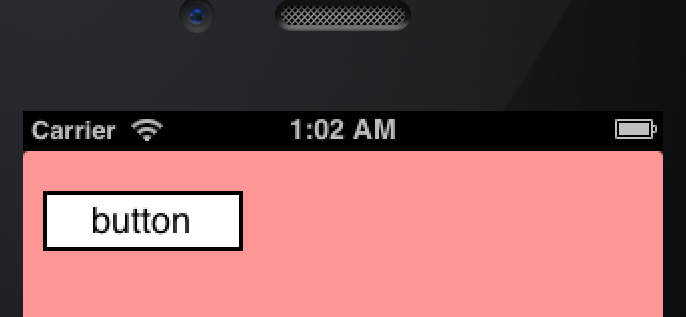
按鈕圖角 Radius Border
要使 Button 有圖角效果,只要設定 button.layer.cornerRadius 即可。
Button with radius border
- button.layer.borderWidth=1.0f;
- button.layer.borderColor=[[UIColor blackColor] CGColor];
- button.layer.cornerRadius = 10;
- [view addSubview:button];
DEMO
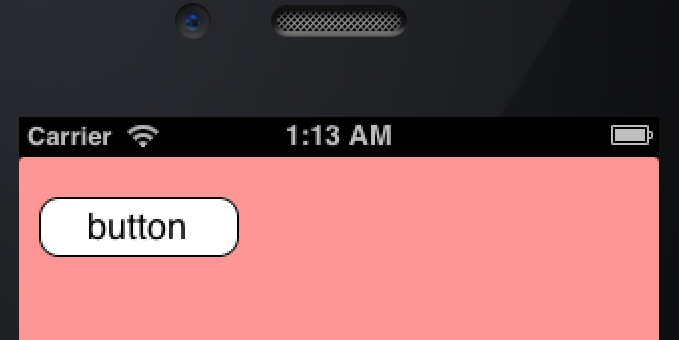
按鈕陰影 Shadow
- button.layer.shadowOffset 設定 x, y 平移量,當 CGSizeMake 第一個值 > 0 ,陰影會往右移動,反之則往左移動,第二個值 > 0 ,陰影會往下移動,反之則往上移動。
- Opacity 陰影透明度,預設是 0 ,所以一定要給這個值,否則看不到陰影效果!!
Button Shadow
- button.layer.shadowOpacity = 0.5;
- button.layer.shadowColor = [[UIColor blackColor] CGColor];
- button.layer.shadowOffset = CGSizeMake(3.0, 3.0);
- button.layer.shadowRadius = 5;
DEMO
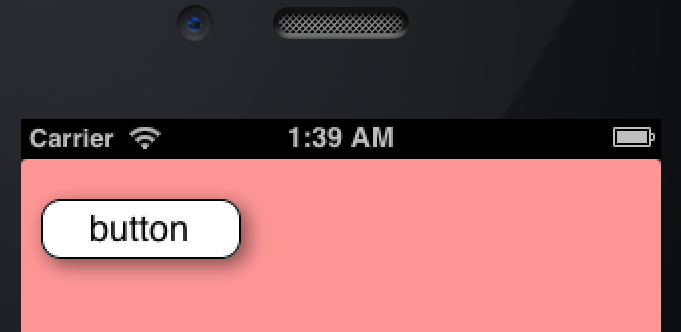
為按鈕綁定點擊事件 ( Click )
在綁定 Click 事件之前, 我們必須先建立一個 function 來處理點擊後的行為,宣告的方式 => (void)Action:(UIButton *)sender, 這只是一個範例, Action 是指事件的名稱。
我建立一個簡單的 function ,當點擊按鈕後,會印出一句 log 訊息。
Clicked Function
- - (void) handleButtonClicked:(id)sender {
- NSLog(@"button have been clicked.");
- }
接著我要綁定點事件給 button ,統共有三個屬性必須設定:
- addTarget
- action : 指定執行的 function
- forControlEvents : 指定事件型態,如 TouchUp, TouchDown ...等
Example
- [button addTarget:self
- action:@selector(handleButtonClicked:)
- forControlEvents:UIControlEventTouchUpInside
- ];
一個簡單的按鈕事件這樣就寫完了,當你點擊按鈕後,程式就會印出 「button have been clicked.」。
回應 (Leave a comment)